《assembly language for the ibm-pc second edition P623》
作者 | 编者 |
---|---|
出版 | 未查询到或未知 |
参考页数 | |
出版时间 | 没有确切时间的资料 目录预览 |
ISBN号 | 无 — 求助条款 |
PDF编号 | 820576358(仅供预览,未存储实际文件) |
求助格式 | 扫描PDF(若分多册发行,每次仅能受理1册) |
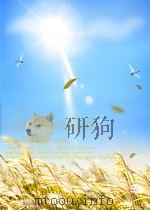
1 Introduction1
1.1Introducing Assembly Language1
Assembly Language Applications3
Machine Language3
1.2Data Representation4
Binary Numbers4
Converting Binary to Decimal7
Hexadecimal Numbers7
Signed Numbers8
Character Storage9
1.3Assembly Language:An Introduction10
Assembly Language Instructions10
A Sample Program11
DEBUG Commands13
The PAGE.COM Program14
1.4Basic Elements of Assembly Language15
Constant15
Statement17
Name18
1.5 Sample HELLO Program19
1.6 Review Questions20
2 Hardware and Software Architecture22
2.1Components of a Microcomputer22
Video Display22
Keyboard23
Disk Drives23
System Unit23
Intel Microprocessor Family25
2.2System Architecture26
Central Processing Unit(CPU)26
Registers28
Flags31
Stack32
Instruction Execution Cycle35
2.3System Software and Memory35
Memory Architecture35
DOS Initialization36
Video Display37
Read-Only Memory(ROM)38
Address Calculation38
Memory Addressing Using Registers39
2.4 Review Questions39
2.5 Programming Exercises41
3Assembly Language Fundamentals46
3.1Data Definition Directives46
Define Byte(DB)47
Define Word(DW)49
Define Doubleword(DD)51
DUP Operator51
3.2Data Transfer Instructions52
MOV Instruction52
Offsets53
XCHG Instruction55
Stack Operations55
3.3 Arithmetic Instructions56
INC and DEC Instructions57
ADD Instruction57
SUB Instruction58
Flags Affected by ADD and SUB58
3.4 Addressing Modes60
Register Operand61
Immediate Operand61
Direct Operand61
Indirect Operand62
Based and Indexed Operands63
Base-Indexed Operand64
Base-Indexed with Displacement64
Summing a List of Numbers65
3.5Program Structure66
Memory Models67
3.6 Review Questions69
3.7 Programming Exercises73
4The Macro Assembler76
4.1The Assembly Process76
A Sample Program77
Assemble the Program78
Link and Run the Program79
4.2Related Files80
Listing File80
Map File81
Batch Files82
4.3Equates83
The Equal-Sign Directive83
EQU Directive84
4.4Operators and Expressions85
Arithmetic Operators85
Boolean Operators86
OFFSET,PTR,and LABEL86
Operands with Displacements88
Other Assembler Operators89
4.5Transfer-of-Control Instructions91
JMP Instruction91
LOOP Instruction93
4.6 Using the 80386 Processor95
4.7Debugging Workshop96
Operand Sizes and Addressing Errors97
4.8 Review Questions98
4.9 Programming Exercises103
5Input-Output Services106
5.1Procedures107
PROC and ENDP Directives107
Sample Program:SUBS.ASM107
Near and Far Procedures108
5.2Software Interrupts111
INT Instruction113
Device Names115
5.3DOS Function Calls116
01h:Console Input With Echo117
02h:Character Output117
05h:Printer Output117
06h:Direct Console Input-Output118
07h:Direct Console Input119
08h:Console Input Without Echo119
09h:String Output119
0Ah:Buffered Console Input119
0Bh:Get Console Input Status121
0Ch:Clear Input Buffer,Invoke Input Function121
BIOS-Level Keyboard Input(INT 16h)121
ASCII Control Characters123
5.4BIOS-Level Video Control(INT 10h)123
Displays,Modes,and Attributes124
127h:Set Video Mode127
01h:Set Cursor Lines128
02h:Set Cursor Position129
03h:Get Cursor Position130
05h:Set Video Page130
06h,07h:Scroll Window Up or Down131
08h:Read Character and Attribute132
09h:Write Character and Attribute133
0Ah:Write Character133
0Fh:Get Video Mode133
11h:Load Default ROM Fonts134
5.5 Review Questions134
5.6 Programming Exercises137
6Conditional Processing141
6.1Boolean and Comparison Instructions141
The Flags Register141
AND Instruction142
OR Instruction144
XOR Instruction145
NOT Instruction146
NEG Instruction146
TEST Instruction147
CMP Instruction147
6.2Conditional Jumps148
Conditional Jump Instruction149
Applications Using Conditional Jumps151
6.3Conditional Loops157
LOOPZ(LOOPE) Instruction157
LOOPNZ(LOOPNE) Instruction158
6.4High-Level Logic Structures159
IF Statement160
WHILE Structure161
REPEAT!!UNTIL Structure163
CASE Structure164
Offset Table165
6.5 Review Questions166
6.6 Programming Exercises169
7Arithmetic172
7.1Shift and Rotate Instructions173
SHL Instruction173
SHR Instruction175
SAL and SAR Instructions176
ROL Instruction176
ROR Instruction177
RCL and RCR Instructions178
7.2Sample Applications179
Shifting Multiple Bytes179
Multiplication and Division180
Display a Number in ASCⅡ Binary181
Isolate a Bit String182
7.3Multiple Addition and Subtraction183
ADC Instruction183
SBB Instruction185
7.4 Signed Arithmetic186
7.5Multiplication and Division187
MUL and IMUL Instructions187
DIV and IDIV Instructions189
Divide Overflow190
7.6ASCⅡ Arithmetic191
AAA Instruction193
AAS Instruction195
AAM Instruction196
AAD Instruction197
7.7Packed Decimal Arithmetic197
DAA Instruction198
DAS Instruction198
BCD Addition Example198
7.8 Review Questions201
7.9 Programming Exercises204
8Numeric Conversions and Libraries208
8.1Character Translation Using XLAT209
The XLAT Instruction209
Character Filtering210
Character Encoding210
8.2Binary to ASCⅡ Conversion213
The WRITEINT Procedure214
8.3ASCⅡ to Binary Conversion218
The READINT Procedure218
8.4 Separately Assembled Modules222
EXTRN Directive223
PUBLIC Directive224
Calling DISPLAYSTR from Another Module224
8.5 Creating External Subroutines226
Individual Subroutine Descriptions227
A Test Program236
More About the Linker237
8.6Stack Parameters238
Recursion239
8.7 Review Questions242
8.8 Programmming Exercises244
9String Processing251
9.1 String Storage Methods252
9.2String Primitive Instructions253
MOVS(Move String)256
CMPS(Compare Strings)258
SCAS(Scan String)260
STOS(Store in String)262
LODS(Load String)263
9.3A Library of String Routines263
READSTRING Procedure264
STRCHR Procedure265
STRCOMP Procedure266
STRCOPY Procedure267
STRDEL Procedure268
STRLEN Procedure269
STRSTR Procedure270
STRUPR Procedure271
WRITESTRING Procedure272
9.4 Creating a Link Library272
9.5 Application: String Library Demo Program275
9.6 Review Questions278
9.7 Programming Exercises280
10Macros and Structures285
10.1Introduction285
Declaring and Calling Macros287
Passing Parameters288
Nested Macros290
LOCAL Directive291
10.2Special Techniques292
Macros Calling Procedures292
Conditional-Assembly Directives293
EXITM Directive295
10.3 Macro Operators297
10.4 A Macro Library299
10.5Advanced MACRO Usage303
Defining Repeat Blocks303
Additional Tips307
10.6Advanced Operators and Directives313
Type Operators313
STRUC Directive314
RECORD Directive316
10.7 Review Questions320
10.8 Programming Exercises323
11Disk Storage327
11.1Disk Storage Fundamentals328
Physical and Logical Chacteristics328
Types of Disks329
Disk Formats330
Disk Directory331
Directory Format332
Sample Disk Directory334
File Allocation Table(FAT)335
Reading and Writing Disk Sectors336
11.2 Application: Sector Display Program337
11.3 Application: Cluster Display Program340
11.4 System-Level File Functions345
DOS Error Codes345
Displaying DOS Error Messages346
DOS Error Message Handler347
File Specifications350
11.5Reading the DOS Command Tail350
11.6Drive and Directory Manipulation352
Set Default Disk Drive(0Eh)353
Get Default Disk Drive(19h)353
Get Disk Free Space(36h)353
Get Current Directory Path(47h)354
Set Current Directory(3Bh)354
Create Subdirectory(39h)355
Remove Subdirectory(3Ah)355
Get Device Parameters(44h)355
11.7File Manipulation357
Get/Set File Attribute(43h)357
Delete File(41h)359
Rename File(56h)360
Get/Set File Date and Time(57h)360
Find First Matching File(4Eh)361
Find Next Matching File(4Fh)362
Set Disk Transfer Address(1Ah)362
11.8 Application:Display Filenames and Dates363
11.9 Review Questions366
11.10 Programmming Exercises368
12File Processing373
12.1Standard DOS File Functions374
Create File(3Ch)374
Open File(3Dh)376
Close File Handle(3Eh)377
Read from File or Device(3Fh)377
Write to File or Device(40h)378
12.2 Application:Create a Text File379
12.3Application:List a Text File383
The File Listing Program385
12.4Application:Display a Student Information File388
Fixed-Length Record Processing388
The Student Information File389
The Student Information Program390
12.5Random File Access395
Move File Pointer(42h)396
12.6Indexing the Student Information File398
The Student Index Program398
12.7 Review Questions406
12.8 Programmming Exercises408
13High-Level Linking412
13.1 General Conventions412
13.2Linking to Turbo Pascal414
Sample Procedure415
Passing Arguments by Value418
Passing Arguments by Reference420
Example:The COUN_CHAR Function421
Identifiers Declared in the Pascal Module423
13.3Turbo Built-In Assembler(BASM)426
Turbo Pascal BASM Syntax426
13.4 Inline Statements and Directives429
13.5Linking to Turbo C431
Compiling and Linking431
Segment Names and Calling Conventions432
Calling the ADDEM Function433
File Encryption Example435
13.6 Review Questions437
13.7 Programming Exercises439
14Advanced Topics Ⅰ444
14.1Completing the Instruction Set444
Loading Pointers445
Indirect Jumps and Calls446
Loading Far Pointers447
Interrupt Control Instructions449
Processor Control Instructions450
Accessing Input-Output Ports451
Flag Manipulation Instructions453
14.2 Defining Segments454
The SEGMENT and ENDS Directives455
The ASSUME Directive458
Segment Override Operator460
Combining Segments460
The GROUP Directive461
Simplified Segment Directives464
14.3Running Programs Under DOS465
COM Programs466
EXE Programs467
Memory Models468
Configuring CONFIG.SYS469
14.4 Review Questions470
14.5 Programming Exercises473
15Advanced Topics Ⅱ477
15.1System Hardware477
Real-Time Clock478
CPU478
Calculating Instruction Timings480
Loop Example482
Loop Example in Pascal484
15.2Instruction Encoding485
Single-Byte Instructions486
Immediate Operands486
Register-Mode Instructions487
Memory-Mode Instructions488
15.3Dynamic Memory Allocation490
Modify Memory Blocks492
Allocate Memory492
Release Allocated Memory493
15.4Interrupt Handling493
Replacing Interrupt Vectors496
Memory-Resident Programs497
Application:The NO_RESET Program498
15.5Defining Real Numbers503
Define Doubleword(DD)504
Define Quadword(DQ)504
Define Tenbyte(DT)505
15.6Intel 80×87 Math Coprocessor505
Instruction Formats506
Evaluating an Expression508
Application:Payroll Calculation Program509
15.7 Review Questions513
15.8 Programmming Exercises514
Appendixes519
A.Binary and Hexadecimal Tutorial519
B.Using DEBUG533
C.Microsoft CodeView547
D.Borland Turbo Debugger555
E.Guide to the Companion Diskette562
F.MASM/TASM Reserved Words566
G.BIOS and DOS Interrupts569
H.Intel 8086/8088 Instruction Set579
I.Answers to Selected Review Questions603
Index617
《assembly language for the ibm-pc second edition P623》由于是年代较久的资料都绝版了,几乎不可能购买到实物。如果大家为了学习确实需要,可向博主求助其电子版PDF文件。对合法合规的求助,我会当即受理并将下载地址发送给你。
高度相关资料
-
- Serious Programming for the IBM PC/XT/AT
- 1985 TAB BOOKS Inc
-
- Excel macros for the IBM PC
- 1990 Windcrest Books
-
- PS/2-PC ASSEMBLY LANGUAGE
- 1989 BRADY NEW YORK
-
- REFERENCE ENCYCLOPEDIA FOR THE IBM PERSONAL COMPUTER SECOND EDITION
- 1983 ASHTON TATE
-
- PC ARCHITECTURE FROM ASSEMBLY LANGUAGE TO C
- 1998 PRENTICE HALL
-
- Assembly Language Programming For The Atari Computers
- 1984 McGraw-Hill Book Company
-
- Structured Programming in Assembly Language For The IBM PC
- 1988 PWS-KENT Publishing Company
-
- Using the IBM Personal Computer:Organization and Assembly Language Programming
- 1984 CBS College Publishing
-
- COMPUTER GRAPHICS FOR THE IBM PC
- 1987 JOHN WILEY & SONS
-
- ASSEMBLY LANGUAGE PROGRAMMING FOR THE 80386
- 1990 MCGRAW-HILL PUBLISHING COMPANY
提示:百度云已更名为百度网盘(百度盘),天翼云盘、微盘下载地址……暂未提供。➥ PDF文字可复制化或转WORD