《DATA STRUCTURES AND ALGORITHMS》
作者 | ALFRED V.AHO JOHN E.HOPCROFT 编者 |
---|---|
出版 | ADDISON-WESLEY PUBLISHING COMPANY |
参考页数 | 427 |
出版时间 | 1983(求助前请核对) 目录预览 |
ISBN号 | 0201000237 — 求助条款 |
PDF编号 | 813266898(仅供预览,未存储实际文件) |
求助格式 | 扫描PDF(若分多册发行,每次仅能受理1册) |
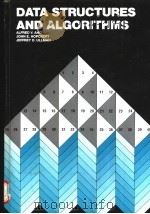
Chapter 1 Design and Analysis of Algorithms1
1.1 From Problems to Programs1
1.2 Abstract Data Types10
1.3 Data Types, Data Structures, and Abstract Data Types13
1.4 The Running Time of a Program16
1.5 Calculating the Running Time of a Program21
1.6 Good Programming Practice27
1.7 Super Pascal29
Chapter 2 Basic Data Types37
2.1 The Data Type “List37
2.2 Implementation of Lists40
2.3 Stacks53
2.4 Queues56
2.5 Mappings61
2.6 Stacks and Recursive Procedures64
Chapter 3 Trees75
3.1 Basic Terminology75
3.2 The ADT TREE82
3.3 Implementations of Trees84
3.4 Binary Trees93
Chapter 4 Basic Operations on Sets107
4.1 Introduction to Sets107
4.2 An ADT with Union, Intersection, and Difference109
4.3 A Bit-Vector Implementation of Sets112
4.4 A Linked-List Implementation of Sets115
4.5 The Dictionary117
4.6 Simple Dictionary Implementations119
4.7 The Hash Table Data Structure122
4.8 Estimating the Efficiency of Hash Functions129
4.9 Implementation of the Mapping ADT135
4.10 Priority Queues135
4.11 Implementations of Priority Queues138
4.12 Some Complex Set Structures145
Chapter 5 Advanced Set Representation Methods155
5.1 Binary Search Trees155
5.2 Time Analysis of Binary Search Tree Operations160
5.3 Tries163
5.4 Balanced Tree Implementations of Sets169
5.5 Sets with the MERGE and FIND Operations180
5.6 An ADT with MERGE and SPLIT189
Chapter 6 Directed Graphs198
6.1 Basic Definitions198
6.2 Representations for Directed Graphs199
6.3 The Single-Source Shortest Paths Problem203
6.4 The All-Pairs Shortest Path Problem208
6.5 Traversals of Directed Graphs215
6.6 Directed Acyclic Graphs219
6.7 Strong Components222
Chapter 7 Undirected Graphs230
7.1 Definitions230
7.2 Minimum-Cost Spanning Trees233
7.3 Traversals239
7.4 Articulation Points and Biconnected Components244
7.5 Graph Matching246
Chapter 8 Sorting253
8.1 The Internal Sorting Model253
8.2 Some Simple Sorting Schemes254
8.3 Quicksort260
8.4 Heapsort271
8.5 Bin Sorting274
8.6 A Lower Bound for Sorting by Comparisons282
8.7 Order Statistics286
Chapter 9 Algorithm Analysis Techniques293
9.1 Efficiency of Algorithms293
9.2 Analysis of Recursive Programs294
9.3 Solving Recurrence Equations296
9.4 A General Solution for a Large Class of Recurrences298
Chapter 10 Algorithm Design Techniques306
10.1 Divide-and-Conquer Algorithms306
10.2 Dynamic Programming311
10.3 Greedy Algorithms321
10.4 Backtracking324
10.5 Local Search Algorithms336
Chapter 11 Data Structures and Algorithms for External Storage347
11.1 A Model of External Computation347
11.2 External Sorting349
11.3 Storing Information in Files361
11.4 External Search Trees368
Chapter 12 Memory Management378
12.1 The Issues in Memory Management378
12.2 Managing Equal-Sized Blocks382
12.3 Garbage Collection Algorithms for Equal-Sized Blocks384
12.4 Storage Allocation for Objects with Mixed Sizes392
12.5 Buddy Systems400
12.6 Storage Compaction404
Bibliography411
Index419
1983《DATA STRUCTURES AND ALGORITHMS》由于是年代较久的资料都绝版了,几乎不可能购买到实物。如果大家为了学习确实需要,可向博主求助其电子版PDF文件(由ALFRED V.AHO JOHN E.HOPCROFT 1983 ADDISON-WESLEY PUBLISHING COMPANY 出版的版本) 。对合法合规的求助,我会当即受理并将下载地址发送给你。
高度相关资料
-
- FINITE ALGORITHMS IN OPTIMIZATION AND DATA ANALYSIS
- 1985 JOHN WILEY & SONS
-
- DATA STRUCTURES AND PROGRAMMING TECHNIQUES
- 1977 PRENTICE-HALL
-
- Data structures and algorithms with object-oriented design patterns in C++
- 1999 John Wiley Sons
-
- DATA STRUCTURES AND ALGORITHMS
- 1983 ADDISON-WESLEY PUBLISHING COMPANY
-
- PROGRAM DESIGN AND DATA STRUCTURES IN PASCAL
- 1986 WADSWORTH PUBLISHING COMPANY
-
- DATA STRUCTURES,COMPUTER GRAPHICS,AND PATTERN RECOGNITION
- 1977 ACADEMIC PRESS
-
- Data Structures and Algorithms 2:Graph Algorithms and NP-Completeness
- 1984 Springer-Verlag
-
- Data Structures and Network Algorithms
- 1983 Society for Industrial and Applied Mathematics
提示:百度云已更名为百度网盘(百度盘),天翼云盘、微盘下载地址……暂未提供。➥ PDF文字可复制化或转WORD